Content |
Literate Programming
If you look at the source code of this page you will notice that there are no :import: directives. So no C++ code gets imported. Instead C++ code gets created from this document. It also creates and executes some gnuplot scripts.
So if you read the source code of this page think of it as a single entity. Don't distinguish between doc-file, C++ code, shell script, gnuplot script. Seeing things this way follows ideas from literate programming.
Producing some Data
The following C++ code will be used later to create data for plotting the \(\sin\) function. Note that the C++ code is not getting imported from some external source. Instead the C++ code is part of the document source. This is similar to how you would embed C++ listings in a \(\LaTeX\) file.
#include <iostream>
const int N = 100;
int
main()
{
for (int i=0; i<=N; ++i) {
const double x = 2*M_PI*i/N;
std::cout << x << " " << sin(x) << std::endl;
}
}
Compile this code from the command line with
$shell> cd tutorial $shell> clang++ -Wall -o sin sin.cc
and then execute it with
$shell> cd tutorial $shell> ./sin > data
Note that the output of sin gets redirected into a file named data.
Plotting Data
We will plot the data with gnuplot. The following commands will executed by gnuplot:
set output "sin.png"
plot "data" using 1:2 with lines
Simply call gnuplot from the terminal like that
$shell> cd tutorial $shell> gnuplot sin.gps
This will produce an image named sin.png that looks like that:
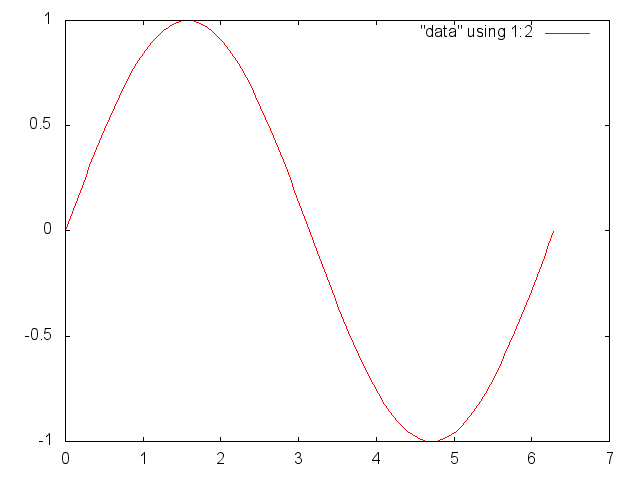
Final Notes
Think of reading some magic book where everything you read actually happens. That's how doctool reads the pagesource.