ULM Generator (Part 4): Fetch/Store Instructions
Material: Instruction Set and “hello, world!” Program
Here the final instruction set that was created:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 | RRR (OP u 8) (X u 8) (Y u 8) (Z u 8)
J26 (OP u 8) (XYZ j 24)
U16R (OP u 8) (XY u 16) (Z u 8)
0x01 RRR
ulm_halt(ulm_regVal(X));
0x02 RRR
ulm_setReg(ulm_readChar() & 0xFF, X);
0x03 RRR
ulm_printChar(ulm_regVal(X) & 0xFF);
0x04 J26
ulm_unconditionalRelJump(XYZ);
0x05 RRR
ulm_sub64(X, ulm_regVal(Y), Z);
0x06 J26
ulm_conditionalRelJump(ulm_statusReg[ULM_ZF] == 0, XYZ);
0x07 J26
ulm_conditionalRelJump(ulm_statusReg[ULM_ZF] == 1, XYZ);
0x08 U16R
ulm_setReg(XY, Z);
0x09 RRR
ulm_fetch64(0, X, 0, 0, ULM_ZERO_EXT, 1, Z);
0x0A RRR
ulm_add64(X, ulm_regVal(Y), Z);
0x0B RRR
ulm_mul64(ulm_regVal(X), ulm_regVal(Y), Z);
|
And here the “hello, world!\n” program:
1 2 3 4 5 6 7 8 9 | 08 00 20 01 # addr for first char
09 01 00 02 # fetch a char
05 00 02 00
07 00 00 04 # addr for the halt instr
03 02 00 00
0A 01 01 01
04 FF FF FB # addr for fetching a char
01 00 00 00
68 65 6c 6c 6f 2c 20 77 6f 72 6c 64 21 0a 00
|
Quiz
Build a computer and write program for it to compute the factorial \(n!\) according to the following (not recursive) algorithm described by a flow chart:
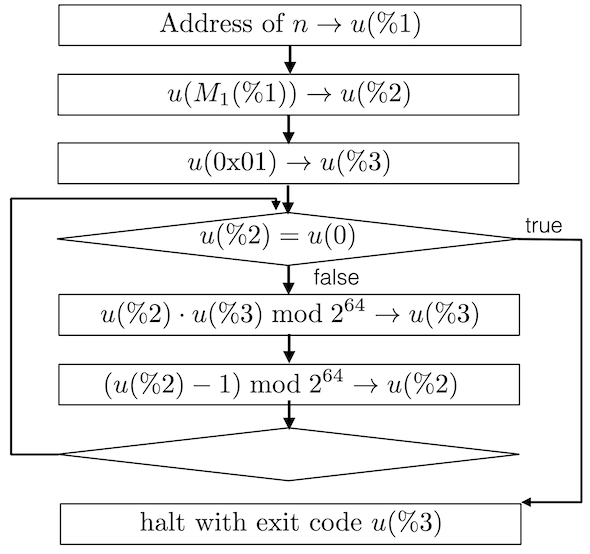
Use the last byte of your program for the variable \(n\). Test your program with differnet values (recall that \(0! = 1\)). Note that the exit code on Unix is truncated to the least significant byte. You can use the debugger to check the actual computed result.
Submit your description of the instruction set isa.txt and your program factorial with submit hpc quiz07 isa.txt factorial.
Browser Test for Flow Charts
For me it is actually easier to generate flow charts with Tikz/Latex. The following chart was created this way and the dvi output converted to a svg (scaleable vector graphic). However, I was told that some browsers have problems to display that corretly (for me it looks ok on Safarai, Google Chrome and Firefox). So let's discuss in class if this works for us: